Toasts
Basic Toasts
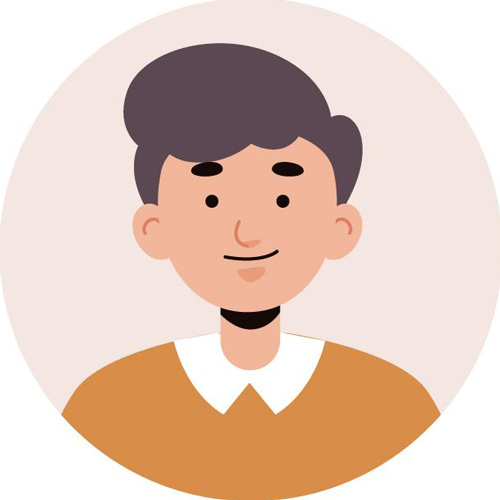
Hello, world! This is a toast message.
<div class="toast fade show border-color" role="alert" aria-live="assertive" aria-atomic="true">
<div class="toast-header border-color">
<img src="assets/images/user/user-1.jpg" class="rounded-circle me-2 wh-32" alt="user-1">
<strong class="me-auto">Alex Smith</strong>
<small>11 mins ago</small>
<button type="button" class="btn-close" data-bs-dismiss="toast" aria-label="Close"></button>
</div>
<div class="toast-body text-body">
Hello, world! This is a toast message.
</div>
</div>
Toast Live Example
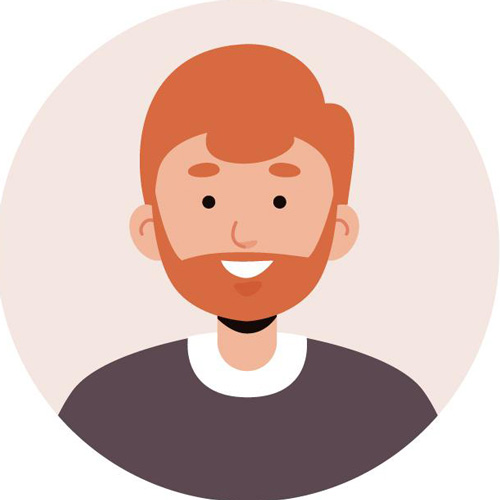
Hello, world! This is a toast message.
<button type="button" class="btn btn-primary" id="liveToastBtn">Show Live Toast</button>
<div class="toast-container position-fixed bottom-0 end-0 p-3">
<div id="liveToast" class="toast border-color" role="alert" aria-live="assertive" aria-atomic="true">
<div class="toast-header border-color">
<img src="assets/images/user/user-2.jpg" class="rounded-circle me-2 wh-32" alt="user-2">
<strong class="me-auto">Luke Ivory</strong>
<small>11 mins ago</small>
<button type="button" class="btn-close" data-bs-dismiss="toast" aria-label="Close"></button>
</div>
<div class="toast-body text-body">
Hello, world! This is a toast message.
</div>
</div>
</div>
<script>
// Show Live Toast
const toastTrigger = document.getElementById('liveToastBtn')
const toastLiveExample = document.getElementById('liveToast')
if (toastTrigger) {
const toastBootstrap = bootstrap.Toast.getOrCreateInstance(toastLiveExample)
toastTrigger.addEventListener('click', () => {
toastBootstrap.show()
})
}
</script>
Toast Translucent
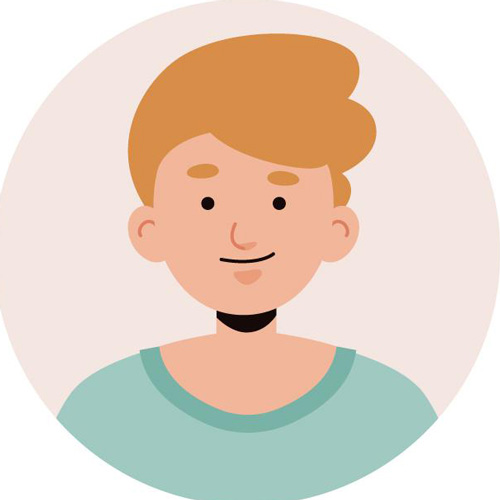
Hello, world! This is a toast message.
<div class="toast fade show border-color" role="alert" aria-live="assertive" aria-atomic="true">
<div class="toast-header border-color">
<img src="assets/images/user/user-3.jpg" class="rounded-circle me-2 wh-32" alt="user-3">
<strong class="me-auto">Andy King</strong>
<small class="text-body-secondary">11 mins ago</small>
<button type="button" class="btn-close" data-bs-dismiss="toast" aria-label="Close"></button>
</div>
<div class="toast-body">
Hello, world! This is a toast message.
</div>
</div>
Stacking Toast
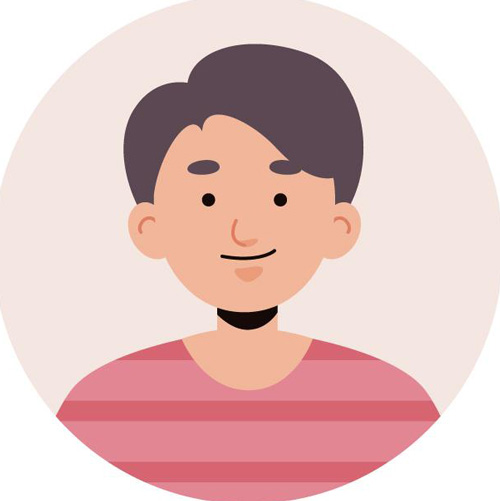
See? Just like this.
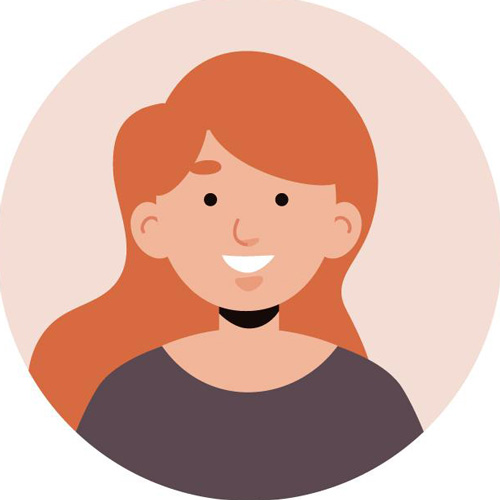
Heads up, toasts will stack automatically
<div class="toast-container position-static">
<div class="toast fade show border-color" role="alert" aria-live="assertive" aria-atomic="true">
<div class="toast-header border-color">
<img src="assets/images/user/user-4.jpg" class="rounded-circle me-2 wh-32" alt="user-4">
<strong class="me-auto">Laurie Fox</strong>
<small class="text-body-secondary">just now</small>
<button type="button" class="btn-close" data-bs-dismiss="toast" aria-label="Close"></button>
</div>
<div class="toast-body text-body">
See? Just like this.
</div>
</div>
<div class="toast fade show border-color" role="alert" aria-live="assertive" aria-atomic="true">
<div class="toast-header border-color">
<img src="assets/images/user/user-5.jpg" class="rounded-circle me-2 wh-32" alt="user-5">
<strong class="me-auto">Ryan Collins</strong>
<small class="text-body-secondary">2 seconds ago</small>
<button type="button" class="btn-close" data-bs-dismiss="toast" aria-label="Close"></button>
</div>
<div class="toast-body text-body">
Heads up, toasts will stack automatically
</div>
</div>
</div>
Custom Content Toast
Hello, world! This is a toast message.
<div class="toast fade show border-color align-items-center" role="alert" aria-live="assertive" aria-atomic="true">
<div class="d-flex">
<div class="toast-body text-body text-body">
Hello, world! This is a toast message.
</div>
<button type="button" class="btn-close me-2 m-auto" data-bs-dismiss="toast" aria-label="Close"></button>
</div>
</div>
Color Schemes Toast
Hello, world! This is a toast message.
<div class="toast fade show border-color align-items-center text-bg-primary border-0" role="alert" aria-live="assertive" aria-atomic="true">
<div class="d-flex">
<div class="toast-body text-white">
Hello, world! This is a toast message.
</div>
<button type="button" class="btn-close btn-close-white me-2 m-auto" data-bs-dismiss="toast" aria-label="Close"></button>
</div>
</div>
Custom Content Button
Hello, world! This is a toast message.
<div class="toast fade show border-color" role="alert" aria-live="assertive" aria-atomic="true">
<div class="toast-body text-body">
Hello, world! This is a toast message.
<div class="mt-2 pt-2 border-top border-color">
<button type="button" class="btn-primary btn-sm">Take action</button>
<button type="button" class="btn-primary btn-secondary btn-sm" data-bs-dismiss="toast">Close</button>
</div>
</div>
</div>